Dependency injection in Angular 2
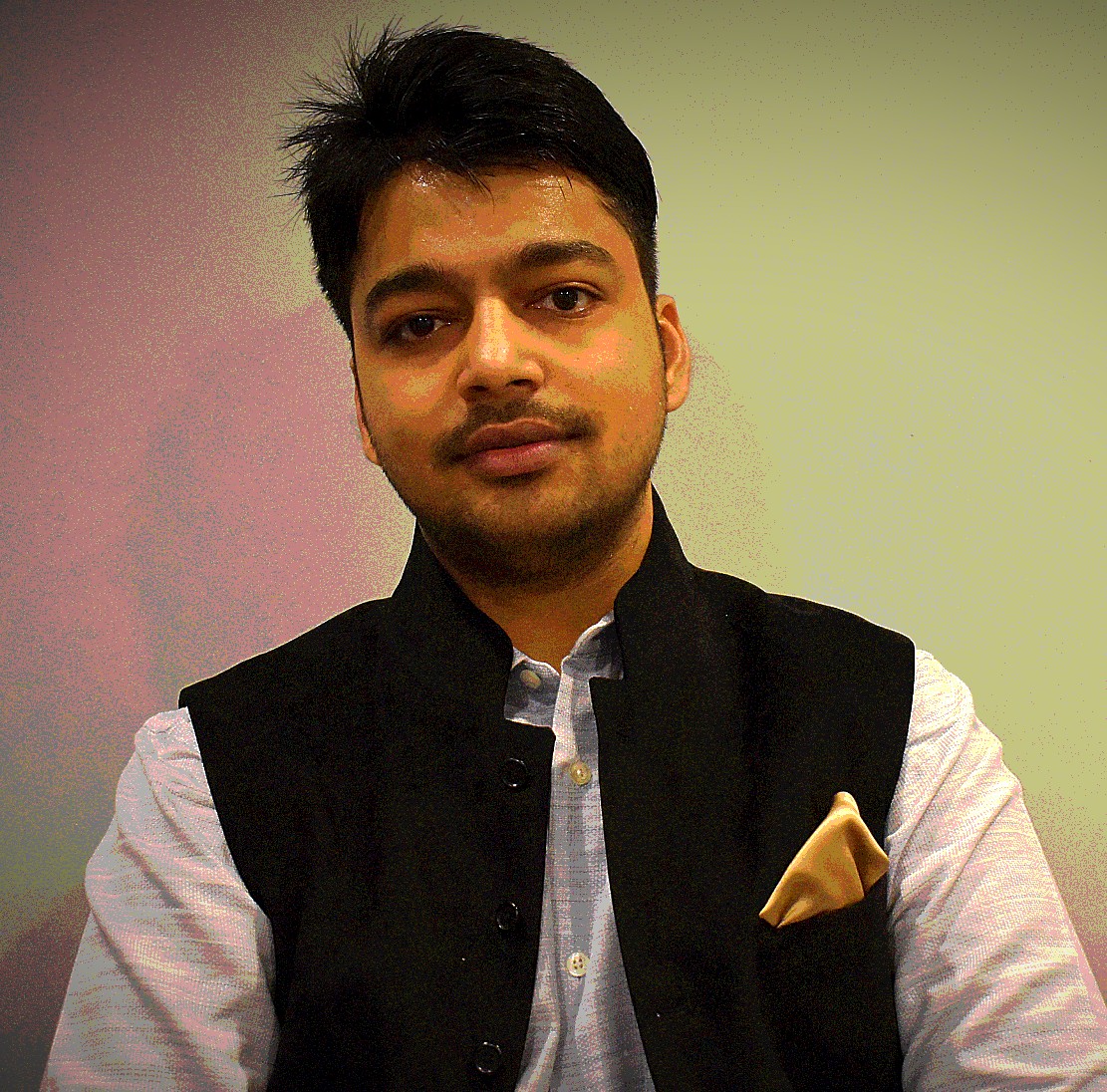
February 7, 2022
f you have some experience with AngularJs, you would know what Dependency injection is and how to use it. Whenever we need some third-party functionality we just inject it. But if you don't know there is nothing to worry as I am going to explain from scratch how it works in Angular 2.
In AngularJs we inject dependencies in module, directives, components etc. But now with Angular 2.x, there is only one way by which we can inject dependency and that is simply by using constructors. Please refer my post where I have explained about constructors in Angular 2.x and have given an overview of dependency injection.
We can inject dependency instead of creating a new instance of them.
Let's understand what it actually means with the help of an example.
Suppose we have a service as shown below:
import { Injectable } from '@angular/core';
@Injectable()
export class ExampleService {
testMethod() {
return 'testing service';
}
}
Now we have a component which is dependent on service declared above. Now here we create a new instance of service in a component as shown below:
class MyComponent {
var mydata = new ExampleService();
console.log(mydata.testMethod();
}
Above we are generating a new instance of service, but it has some drawbacks.One of them is if our service is later dependent on some dependencies it would be really difficult to manage if we have a large app.
So, a better way is to inject them using injector rather than creating a new instance as shown below:
import { Component } from '@angular/core'
import { ExampleService } from './example.service'
@Component({
selector: 'my-component',
templateURL: 'app/component/example.component.html',
providers: [ExampleService]
})
export class MyComponent {
constructor(myService : ExampleService) { }
console.log(myService.testMethod());
}
So we are good with how dependencies are injected into our Angular app. Now, let's suppose we have another component sibling to MyComponent (both components are at the same level):
import { Component } from '@angular/core'
import { ExampleService } from './example.service'
@Component({
selector: 'my--sibling-component',
templateURL: 'app/component/example.sibling.component.html',
providers: [ExampleService]
})
class MySiblingComponent {
constructor(myService : ExampleService) { }
console.log(myService.testMethod());
}
A question is how many instances of ExampleService is created in our app?
The answer is 2 instances reason being two components are siblings to each other so angular creates two instances of the service.
But if we would have a service injected to root component and it has Childs as first (MyComponent) and second (MySiblingComponent) component then angular would have created only 1 instance of the service. i.e. If any changes to service were made in the first component it would reflect in another component as well. Consider an example as shown below:
Let's suppose both MyComponent and MySiblingComponent are the child of AppComponent and we don't have providers in MyComponent and MySiblingComponent. Instead, we have providers defined in AppComponent then only one instance of the service would have been created.
That's all I have to share on how we can inject dependencies in the Angular app. To summarize, in the above post I have explained about dependency injection, constructor, and providers. Subscribe to channel to know about upcoming posts.